MakePlot#
This tutorial is for the MakePlot class available in the TFC utilities. This class is designed to speed up plotting, as well as create pretty, journal-article-ready plots. Please note that this class is designed to be used in regular Python scripts, and the plots may appear scrunched in these Jupyter notebooks. Let’s begin by importing the MakePlot class.
[1]:
import numpy as np
from tfc.utils import MakePlot
# This line is added for Python notebooks but is not necessary in a regular Python script.
%matplotlib inline
The MakePlot class is initializied by specifying the relavent axes labels: the default interpreter for these labels is the TeX interpreter. The x- and y-lables must be specified, and they constitue the only required arguments for the class initialization. Optional keyword parameters allow for the specification of other axes and labels:
zlabs - Optional keyword argument for specifying z-axis labels. Doing so will force the axes to be type Axes3D.
twinYlabs - Optional keyword argument for specifying twin y-labels.
titles - Optional keyword argument for specifying the titles.
For example, suppose we wanted a 3D plot with labels \(x\), \(y\), and \(z\). This could be accomplished using:
[2]:
p = MakePlot(r'$x$',r'$y$',zlabs=r'$z$')
p.show()
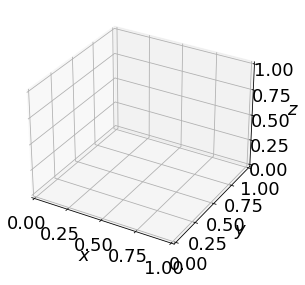
where the show method is used to visualize the plot.
The shape of the labels provided determines how many and where the subplots will be located; the None value is used to specify that a plot does not exist in that location. For example, suppose that we wanted to split the plotting window into a 2x2 array, but we do not want a plot in the bottom-left. Further, suppose that we want twin y-labels for these plots.
[3]:
xlabs = [[r'$x_1$',r'$x_2$'],[None,r'$x_4$']]
ylabs = [[r'$y_1$',r'$y_2$'],[None,r'$y_4$']]
twinYlabs = [[r'twin $y_1$',r'twin $y_2$'],[None,r'twin $y_4$']]
p = MakePlot(xlabs,ylabs,twinYlabs=twinYlabs)
p.FullScreen()
p.show()
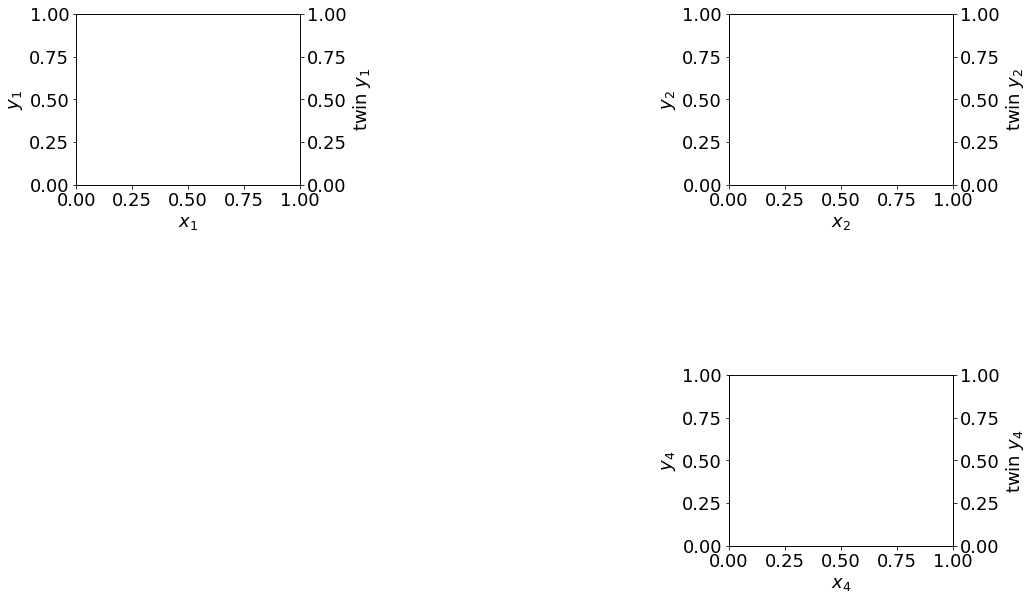
The FullScreen method was used here to increase the size of the plotting window to match the size of the screen.
The MakePlot class provides access to the subfigures and axes that make up the figure via Python lists, named fig and ax respectively; the elements in these lists are ordered from left-to-right and top-to-bottom. For example, suppose we wanted to add a plot to the bottom-right plot in the previous figure. This would correspond to the third element in the axes list.
[4]:
x = np.linspace(0,2*np.pi,100)
p.ax[2].plot(x,np.sin(x))
p.FullScreen()
p.show()
p.fig # This line is added to force plot to display again in the Python notebook. This line is not necessary if using a regular Python script.
[4]:
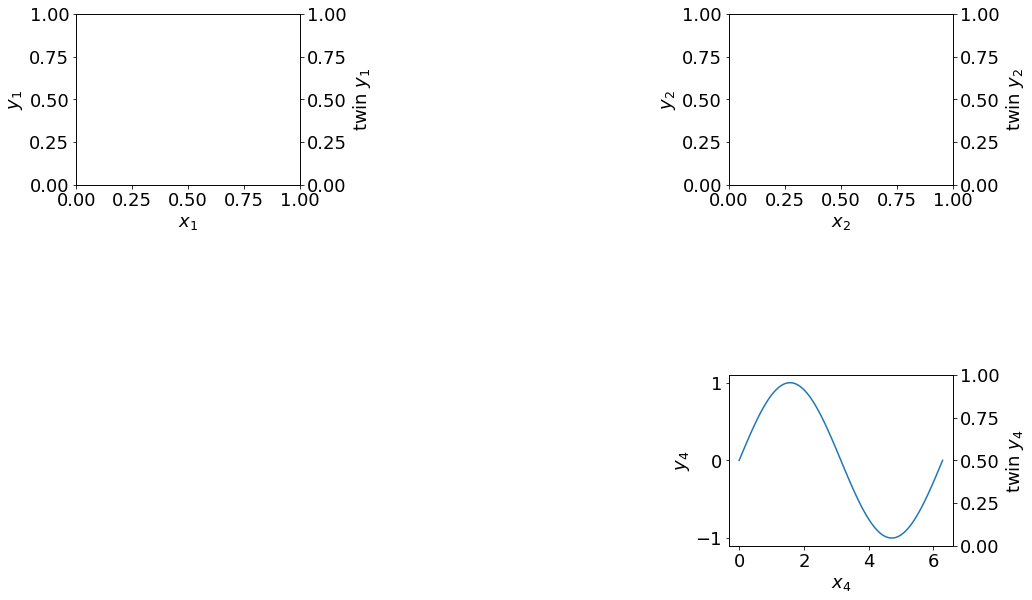
Saving figures#
The MakePlot class offers a few different options for saving the figure:
save - Save the plot as an image.
savePickle - Save the plot using a pickle format so that it can be openend in Python later and further modified if desired.
saveAll - Save the plot both as an image and using the pickle format.
The save option and saveAll option come with two optional keywords:
transparent - This boolean arguement makes the background of the plot transparent. The default is True.
fileType - This optional string argument sets the format of the image. The default is PDF. For a list of options, see the format options for matplotlib’s savefig function.
For example, let’s save the figure as a transparent PDF titled “MyFigure.”
[5]:
p.save("MyFigure")
Miscellaneous#
The MakePlot class also comes equipped with a PartScreen function that can be used to specify the size of the plotting window in inches. For example, let’s create a plot that is 5in x 5in.
[6]:
p = MakePlot('x','y',titles='Test Plot')
p.ax[0].plot(x,np.sin(x))
p.PartScreen(5,5)
p.show()
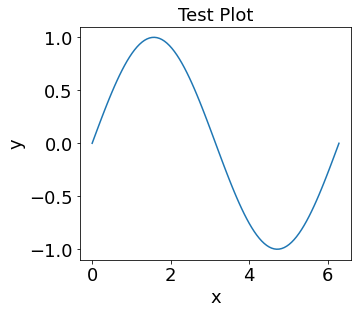